Elementary Concepts of Objects and Classes in Java Programming
Java, known for its versatility and widespread applications, from mobile applications to web development, is considered one of the most popular programming languages. At the core of the object-oriented programming (OOP) pattern in Java lie two fundamental concepts: objects and classes. For anyone who is about to begin their journey in Java programming, these elementary concepts are crucial to understand.
Object-Oriented Programming (OOP)
Object-oriented programming is a programming paradigm that models real-world entities as “objects.” These objects encapsulate both data and behaviour, making programs more modular, reusable, and easier to maintain. Java, as a fully object-oriented language, relies on objects and classes to structure its programs.
1. Definition of OOP
- A programming paradigm based on the concept of “objects,” is known as Object-Oriented Programming. These objects are instances of classes.
- Objects represent real-world entities and their behaviour by combining data (attributes) and methods (functions).
4. Advantages of OOP
- Modularity: Division of code is done into smaller, reusable modules like classes and objects.
- Code Reusability: Inheritance involves reducing duplication and promoting efficient code reuse.
- Scalability: Easier to manage and expand the system based on requirements.
- Security: Encapsulation involves ensuring data security and controlled access.
- Maintainability: Future modifications and debugging are simplified.
5. Real-World Example
- A class Bank Account may have attributes like
accountNumber
,balance
, and methods likedeposit()
,withdraw()
. A unique object of the class represents each customer.
Real-world systems are more naturally modelled in OOP, making it an effective approach for software design and development.
Classes
A class in Java is considered as a template or blueprint to create objects. The behaviours (methods) and properties (attributes) that the objects created from the class will have, is defined by the class. Consider a class as a design plan or recipe which outlines the look of an object and plans what it should be able to do.
1. Definition of a Class
- A class may be defined as a blueprint or template for creating objects.
- The set of properties (attributes) and behaviours (methods) that objects will have are defined by the class.
2. Components of a Class
a. Attributes (Fields/Variables)
- Attributes which may also be termed as fields or variables, represent the data or properties of a class.
- Example: The attributes of a car can be
color
,model
, andspeed
.
b. Methods (Functions)
- Methods which may also be termed as functions define the behaviour or functionality of the class.
- Example: The methods of the car can be
start()
,drive()
, orstop()
.
c. Constructors
- Constructors are special methods used for the initialization of the objects of the class.
- In Java, the constructor and the class have the same name.
- A constructor has no return type.
3. Characteristics of a Class
- Encapsulation: Involves combining data (attributes) and functions (methods) into a single unit.
- Modularity: Classes can be reused and extended, acting as modular units of code.
- Abstraction: A class defines the essential details while hiding the implementation, thus providing abstraction.
6. Types of Classes in Java
a. Concrete Class
- A concrete class is a standard class that can be instantiated to create objects.
b. Abstract Class
- An abstract class is one that cannot be instantiated and is meant to be extended by other classes.
- It contains abstract methods (without implementation).
c. Inner/Nested Class
- An inner or nested class is defined as a class defined inside another class.
d. Static Class
- A static class allows accessing methods and attributes without creating an instance.
7. Access Modifiers for Classes
- These modifiers are used to control the visibility of a class and its members:
- Public: Can be accessed everywhere.
- Default: Can be accessed within the same package.
8. Advantages of Using Classes
- Code reusability is promoted.
- Modularity and readability is enhanced.
- Object-oriented programming principles like inheritance and polymorphism are facilitated.
Classes are fundamental building blocks in object-oriented programming and prove to be every effective in organizing code for large-scale projects.
Syntax of a Class
Here is a basic example of a class in Java:
class Car
{ // Attributes
String brand;
String colour;
int year;
Car(String brand, String color, int year) // Constructor
{
this.brand = brand;
this.color = color;
this.year = year;
}
void displayDetails() // Method
{
System.out.println("Brand: " + brand);
System.out.println("Colour: " + colour);
System.out.println("Year: " + year);
}
}
Output:
Brand: Maruti Suzuki
Colour: Black
Year: 2020
In this example:
- The properties of the object are represented by the attributes mentioned as brand, colour and year.
- The objects of the class are initialized using a special method mentioned as constructor.
- The behaviour of the object is defined by methods mentioned as displayDetails().
Objects
An object is a paradigm of a class. The structure of the program is defined by a class while the actual entity that exists in memory is an object. It can interact with other objects. Objects represent real-world entities, like a specific car, a person, or a bank account.
1. Definition of an Object
- An object is defined as an instance of a class.
- Real-world entity with specific characteristics (attributes) and behaviours (methods) are represented through an object.
2. Characteristics of Objects
a. State
- It is a representation of the data or attributes of the object.
- ExamplE: the state of a Car object could include
color
,model
, andspeed
.
b. Behaviour
- It is representation the actions or methods performed by the object.
- Example: the behaviour of the
Car
object can includedrive()
,stop()
, oraccelerate()
.
c. Identity
- It is a unique identifier for the object in memory.
- Example: Two
Car
objects with the same state but different identities in memory are distinct.
4. Attributes of Objects
- Specific values assigned to the fields of an object are attributes.
- Example:
·
myCar.colour = "Red"; // Assigning value to an object’s attribute
5. Methods in Objects
- Actions or behaviours are performed by methods used in objects.
- Example:
·
myCar.drive(); // Invoking a method on the object
7. Types of Objects
a. Real-world Objects
- These are physical entities, such as a car, a house, or a person.
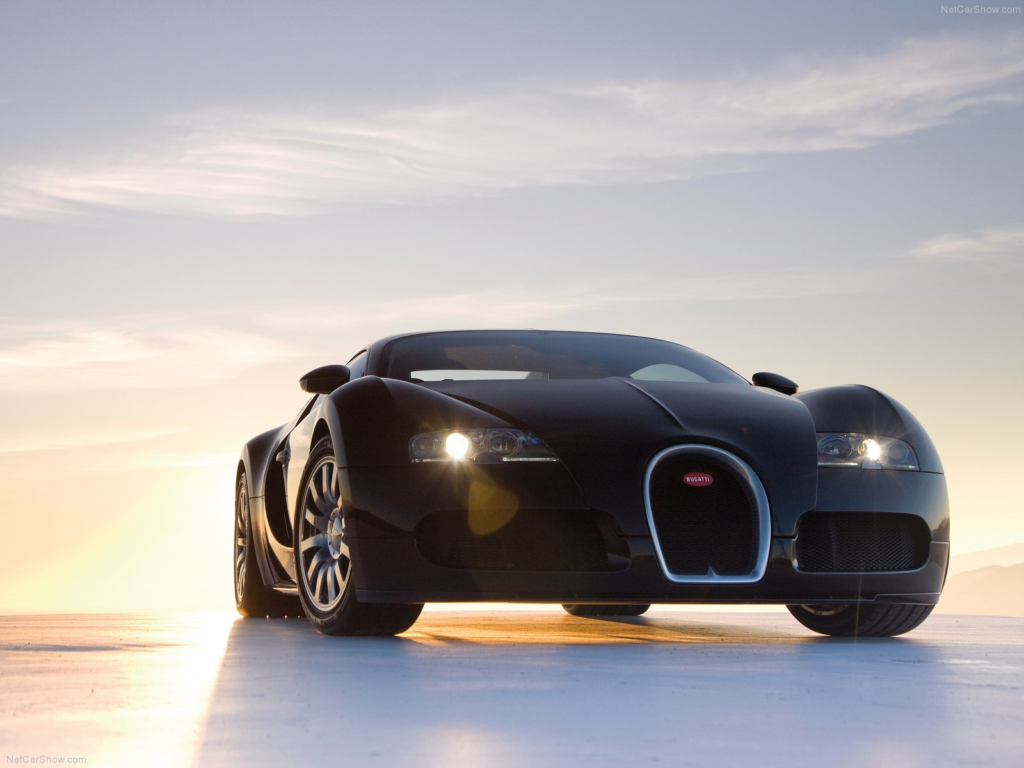
b. Programming Objects
- These are entities in software, like an array, a file or a database connection.
8. Object-Oriented Programming (OOP) Principles Related to Objects
- Encapsulation: Data and methods are encapsulated in objects.
- Inheritance: Objects have the ability to acquire properties and behaviour from parent classes.
- Polymorphism: Different forms, such as having overridden methods can be taken by the objects.
9. Advantages of Objects
- Modularity, where objects break down complex systems into smaller, manageable components.
- Reusability, where objects can be reused across different scenarios.
- Abstraction, where an object hides implementation details, thus simplifying interaction with complex systems.
10. Real-world Example
- Class:
bike
- Objects: A red
bike
with a speed of 80 km/h and a bluebike
with a speed of 90 km/h.
Creating Objects in Java
To create an object in Java, you use the new keyword along with the class constructor. For example:
class Main
{
void main()
{
Car myCar = new Car("Toyota", "Red", 2021); // Creating an object of the Car class
myCar.displayDetails(); // Accessing object methods
}
}
In this example:
- An object of the Car class has been created mentioned as myCar.
- The object is initialized with specific values such as Toyota, Red and 2021 using the constructor Car.
- The method displayDetails() is called on the object to perform an action.
Key Characteristics of Objects and Classes
Encapsulation
Encapsulation is the process of bundling all the data (attributes) and methods (functions), operating into a class which is a single unit. It restricts direct access to some of the components of an object by using protected, public and private access modifiers.
For example:
class BankAccount
{
double balance;
BankAccount(double initialBalance)
{
balance = initialBalance;
}
void deposit(double amount)
{
if (amount > 0)
{
balance += amount;
}
}
double getBalance()
{
return balance;
}
}
Output:
Current Balance: 1500.0
Here, the balance attribute is private, ensuring it cannot be accessed directly. Instead, methods like deposit() and getBalance() provide controlled access.
Inheritance
The mechanism by which the attributes or methods of one class (derived or child class) is inherited by another class (base or parent class). It establishes a hierarchical relationship between classes and promotes the reuse of code.
Example:
class Vehicle
{
String type;
void displayType()
{
System.out.println("Type: " + type);
}
}
class Bike extends Vehicle
{
String brand;
void displayBrand()
{
System.out.println("Brand: " + brand);
}
}
class Main
{
void main()
{
Bike myBike = new Bike();
myBike.type = "Two-Wheeler";
myBike.brand = "Yamaha";
myBike.displayType();
myBike.displayBrand();
}
}
Output:
Type: Two-wheeler
Brand: Yamaha
Polymorphism
The word polymorphism means “many forms.” This mechanism allows objects of different classes to be treated as objects of a common superclass. The processes of method overriding and method overloading are typically used to achieve the principle of polymorphism.
- Method Overloading: This process involves multiple methods with the same name but different parameters.
- Method Overriding: A child class provides a specific implementation for a method in the parent class.
Abstraction
The main focus of Abstraction is hiding implementation details. It shows the essential features of an object only. This principle enables developers to focus on what an object does rather than how it does it, thus reducing complexity and enhancing maintainability.
Example of an abstract class:
abstract class Animal
{
abstract void makeSound();
}
class Dog extends Animal
{
void makeSound()
{
System.out.println("Bark");
}
}
class Main
{
void main()
{
Animal myDog = new Dog();
myDog.makeSound();
}
}
Output:
Bark
Drawing a Heart with a Message Using Python Turtle – Easy Python Project for Kids
We're going to use a fun Python project for kids called Turtle to draw a beautiful red heart, and th…
Create a Fun Animal Facts Website: A Web Development Project for Kids HTML beginners
Are you ready to dive into the exciting world of web development? Whether you’re a kid curious…
Easy MIT App Inventor Project – “Choose Your Favorite Animal” App
MIT App Inventor Project
ICSE 10th String handling most important questions 2026
Most Important String Handling Questions for ICSE Class 10 (2026) Hey there, Class 10 warriors! 👋Are…
70+ CBSE 12th Data Handling Using Pandas – Series Important Questions
70+ CBSE 12th Data Handling Using Pandas – Series Important Questions Boost your Pandas skills…
Python Fundamentals: 20 Operator and Expression in Python to Boost Your Coding Skills
Introduction to Operator and Expression in Python Operators and Expressions are basic and essential …
Explore Our Featured Courses!
CBSE Math Course for Grade 1-8 – Live 1 on 1 session
Master mathematics with our CBSE-aligned Math Course designed for students in Grades 1-8. This cours…
CBSE 12th Computer Science Revision Course
Join us for an exclusive online revision session, where we’ll predict the most expected questi…
Java programming for ICSE 10th
Key Features Comprehensive Syllabus Coverage Interactive Learning Exam-Oriented Approach Beginner-Fr…
App Development for Kids
Empowering Young Minds: MIT App Inventor for Kids This comprehensive 16-hour course introduces kids …
Scratch Programming Course for Complete Beginners
Start Your Scratch Programming Journey Today for FREE! Unlock your creativity and coding potential w…
Find the perfect course
All of our courses are designed to help you learn the fundamentals of Java Programming and ace your exams