Table of Contents
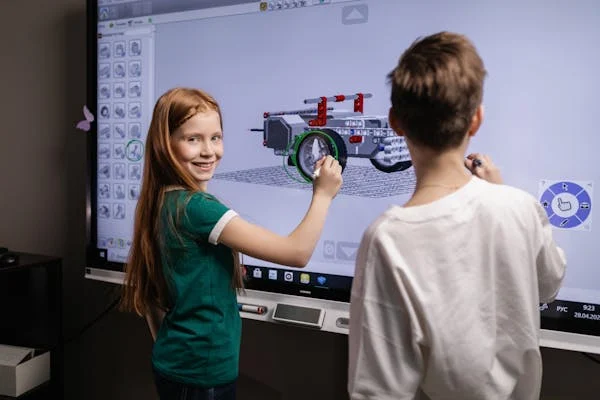
Introduction to Operator and Expression in Python
Operators and Expressions are basic and essential in programming because they allow to perform various operation on data. Operators are the special symbol used in performing operations on variables and values which allows calculations, making logical decisions and for comparison. They serve as the base for expressions, which are important to the most of the programming languages. Understanding various types of functions of operators is important for creating efficient and successful code. By mastering utilization allows you to create complicated expressions that will solve real world issues, control program flow and alter data dynamically. This blog will guide you through understanding deep into various types of operators, their purposes, and their utilization process.
Types of Operators
1. Arithmetic Operator
Arithmetic operators in Python are used to do various basic mathematical operations. Each operators has their own specific role, helps you solve mathematics problem.
Operators | Description | Example | Output |
+ | Addition | 8+5 | 13 |
– | Subtraction | 3-2 | 1 |
* | Multiplication | 9*5 | 45 |
/ | Division | 9/3 | 0 |
// | Floor Division | 10//3 | 3 |
% | Modulus (remainder) | 7%2 | 1 |
** | Exponentiation(power) | 6**2 | 36 |
Let’s understand this by practical:
1. Addition (+): This operator adds two integer or decimal number together. We can use this operator in real-world for adding price in groceries.
Code:
apple = 80
banana = 50
total = apple + banana
print(total)
Output: 130
2. Subtraction (-): These operators subtract one number from another. It can be used in real life to calculate the remaining balance after a withdrawal.
Code:
total_money = 10000
withdrawal = 8000
remaining_balance = total_money - withdrawal
print(remaining_balance)
Output: 2,000
3. Multiplication (*): This operator is used to multiply two numbers on computers. It can be used in real life for calculating the area of a rectangular room where we will multiply this two values (width * length).
Code:
length = 4
breadth = 6
area = length * breadth
print(area)
Output: 24
4. Division(/): This operator is used to divide one number from another number and it will return the quotient as a floating point number. In a real-life scenario, it is dividing the bill among friends.
Code:
total_bill = 500
total_friends = 5
print(total_bill/total_friends)
Output: 100.0
5. Floor Division(//): It is the same as normal division, but the only difference is that it returns the quotient as an integer number. A real-world example would be calculating how many mangoes can fit into fully filled boxes.
Code:
total_mangoes = 525
box_capacity = 20
total_filled_boxes = total_mangoes // box_capacity
print(total_filled_boxes)
Output: The output will be 26, so the total fully filled boxes would be 26.
6. Modulus (%): It is also known as remainder, used for calculating remainders after dividing two numbers. Let’s take an example of the above scenario in which we can find the remaining mangoes left.
Code:
total_mangoes = 525
box_capacity = 20
leftover_mangoes = total_mangoes % box_capacity
print(leftover_mangoes)
Output: The output will be 5, so the leftover mangoes are 5.
7. Exponentiation(**): This operator returns the result of raising the first operand to the power of the second operand. In real-world example, it can be used where multiplication are repeated with same number.
Code:
a = 8
b = 6
total = a**b
print(total)
Output: The output of this program is 262144.
2. Comparison Operator
Comparison operators are used to compare two statements and return output in true or false.
Operator | Description | Example | Output |
== | Equal to | 9 == 9 | True |
!= | Not equal to | 8 != 9 | True |
> | Greater than | 5 > 3 | True |
< | Less than | 2 < 7 | True |
>= | Greater than or equal to | 7 >= 5 | True |
<= | Less than or equal to | 8 <= 8 | True |
1. Equal to (==): This operator check two values if they are same. If it will be same then it will return true if not then it will return false.
Code:
9 == 9
#Output: True
8 == 9
#Ouput: False
2. Not equal to (!=): This operator check two values if the are not same.
Code:
8 != 9
#Output: True
8 != 8
#Output: False
3. Greater than(>): This operator is used to check between two values if the first is one greater than the second.
Code:
5 > 3
#Output: True
3 > 8
#Output: False
4. Less than (<): This operator is very similiar to the mathematical less than operator which checks between two values if the first one is smaller than second digit/string
Code:
2 < 7
#Output: True
7 < 4
#Output: False
5. Greater than or equal (>=): It checks between two values if the first one is greater than the second and also check if the both values are same.
Code:
7 >= 5
#Output: True
7 >= 7
#Output: True
6 >= 2
# Output: False
6. Less than or equal to (<=): It checks between two values if the first one is smaller than the second one and also check if the both values are same.
Code:
6 <= 7
#Output: True
8 <= 8
#Output: True
7 <= 2
#Output: False
3. Logical Operator
Three types of logical operator are there:
1. And Operator: It will check both condition if they are true then only it returns True.
2. Or Operator: It will check both condition and return True if any on of them will be true.
3. Not Operator: It reverse the result, return answer in False if the result is true.
Code:
# Logical operations
a = True
b = False
print(a and b) # Output: False
print(a or b) # Output: True
print(not a) # Output: False
Expression in Python
An expression in Python is a collection of values, variables, and operators which can be evaluated resulting in a single result. Expressions are the fundamental building blocks of any program and also can range from a single value to a complicated combination of values, variables, and operators. Let’s understand this by practical.
Example:
#Simple Expression
10 + 6
Output: 16
#Expression used with variables
a = 6
b = 9
print(a+b)
Output: 15
#Complicated Expression
a = 3
b = 6
c = 2
print(a+b-c*3)
Output: 3
In the third example, Python used precedence rules to solve this problem.
Order of operations in Python
In Python, the order of operations (also referred to as operator precedence) governs the order in which operators are evaluated in an expression. Understanding this sequence helps to avoid misunderstandings when analyzing Python expressions. The precedence rules are comparable to those of mathematics. Python follows the PEMDAS rule, which stands for Parenthesis, Exponents, Multiplication, Division, Addition, and Subtraction.
1. Parentheses: This means anything in parentheses must be solved first.
2. Exponents: It always use for multiplying multiple times and also perform other mathematical operations they are always evaluated from right to left.
3. Multiplication and Division: It basically perform multiplication and division. It always perform from left to right.
4. Addition and Subtraction: It performs addition by adding two values and subtraction by subtracting from the first value to the second. It always perform from left to right.
Example: Evaluate the following 2 * 8 + 5 – 2.
First we will apply PEMDAS rule:
1. Multiplication: 2 * 8 = 16
2. Addition: 16 + 5 = 21
3. Subtraction: 21 – 2 = 19
Result: 19
Questions: Hands-On Practice
Activity 1: Arithmetic Operations
1. Write a Python script to perform the following calculations:
a. Add 15 and 10.
b. Subtract 50 from 158.
c. Multiply 7 by 9.
d. Divide 876 by 7.
Activity 2: Comparison Operations
2. Check if the given statements are true or false.
a. 498 is greater than 365
b. 937 is smaller than 345
c. 9374 is equal to 9374
d. 83 is less than or equal to 83
Activity 3: Logical Operations
3. Write Python code to evaluate these conditions:
a. The negation of 121 > 150
.
b. Both 9 > 6
and 8 == 8
.
c. Either 4 > 8
or 60 < 80
.
Activity 4: Evaluate: 9 + 4 * 3 - 6 /
2
Conclusion
Learning these concepts are crucial in future because these are the topics that will be used very often, so mastering Operator and Expression concepts will make your base strong and help you understand the code more better and you also will be able to write efficient Python code. Practice is the one more thing that make a beginner to advance their level. With these basic concept you can solve sophisticated problem without overwhelming.
In case if you have any doubt or any question you want to ask you can reach us through the comment. Comment down your s answers for us to evaluate !
Drawing a Heart with a Message Using Python Turtle – Easy Python Project for Kids
We're going to use a fun Python project for kids called Turtle to draw a beautiful red heart, and th…
Python Fundamentals: 20 Operator and Expression in Python to Boost Your Coding Skills
Introduction to Operator and Expression in Python Operators and Expressions are basic and essential …
Transform Your Child: Best 8-Week Python Coding Course for Kids!
Whether you're dreaming of creating games, coding cool apps, or diving into the world of artificial …
Top 5 Amazing Mini Python Projects for kids
Why Python Projects for kids? Have you ever wanted to create cool and crazy things on your computer?…
CBSE Math Course for Grade 1-8 – Live 1 on 1 session
Master mathematics with our CBSE-aligned Math Course designed for students in Grades 1-8. This cours…
CBSE 12th Computer Science Revision Course
Join us for an exclusive online revision session, where we’ll predict the most expected questi…
Java programming for ICSE 10th
Key Features Comprehensive Syllabus Coverage Interactive Learning Exam-Oriented Approach Beginner-Fr…
App Development for Kids
Empowering Young Minds: MIT App Inventor for Kids This comprehensive 16-hour course introduces kids …
Scratch Programming Course for Complete Beginners
Start Your Scratch Programming Journey Today for FREE! Unlock your creativity and coding potential w…
Find the perfect course
All of our courses are designed to help you learn the fundamentals of writing and other related topics.
Leave a Comment